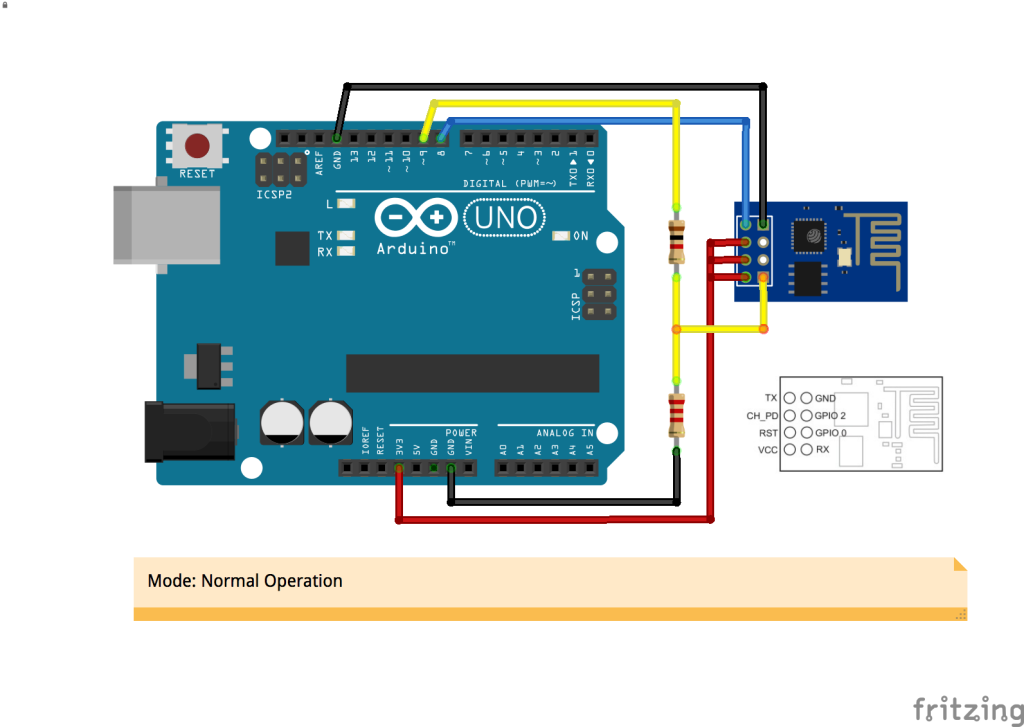
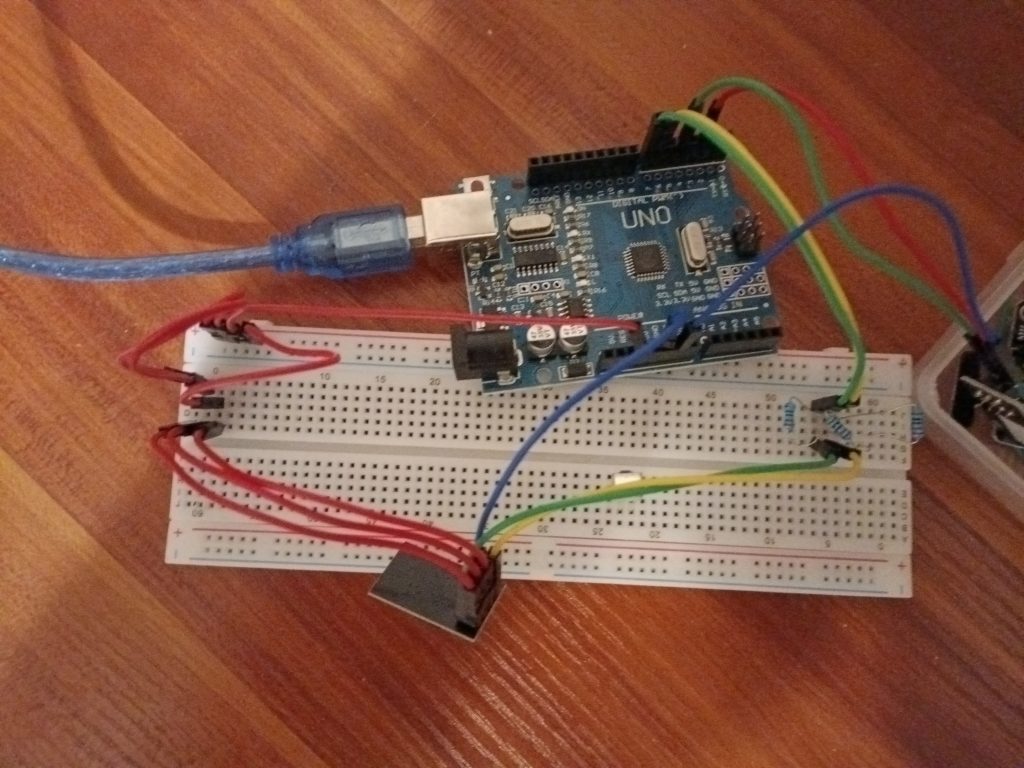
Для начала нужно сменить через AT-команду baud rate самом модуле..
/*
Software serial multiple serial test
Receives from the hardware serial, sends to software serial.
Receives from software serial, sends to hardware serial.
The circuit:
* RX is digital pin 10 (connect to TX of other device)
* TX is digital pin 11 (connect to RX of other device)
Note:
Not all pins on the Mega and Mega 2560 support change interrupts,
so only the following can be used for RX:
10, 11, 12, 13, 50, 51, 52, 53, 62, 63, 64, 65, 66, 67, 68, 69
Not all pins on the Leonardo and Micro support change interrupts,
so only the following can be used for RX:
8, 9, 10, 11, 14 (MISO), 15 (SCK), 16 (MOSI).
created back in the mists of time
modified 25 May 2012
by Tom Igoe
based on Mikal Hart's example
This example code is in the public domain.
*/
//#include <ArduinoJson.h>
#include <SoftwareSerial.h>
SoftwareSerial mySerial(6, 7); // RX, TX
void setup() {
// Open serial communications and wait for port to open:
Serial.begin(9600);
while (!Serial) {
; // wait for serial port to connect. Needed for native USB port only
}
// set the data rate for the SoftwareSerial port
mySerial.begin(19200);
// mySerial.println("AT+IPR=115200");
Serial.println("~~~Write data~~~");
mySerial.println("AT+UART_DEF=9600,8,1,0,0");
Serial.write(mySerial.read());
}
void loop() { // run over and over
if (mySerial.available()) {
Serial.write(mySerial.read());
}
if (Serial.available()) {
mySerial.write("AT");
}
}
Выше пример. Измените 6 и 7 под себя… На RX и TX пины обязательно резисторы.. Модуль работает на 3.3в.. У меня стоит 10кОм
ЕСП модуль.. желательно питать из внешнего источника питания
Ниже пример метеостанции на арБуино с модулем DHT11 и ESP8266
#include "DHT.h"
#include <ArduinoJson.h>
#include <WiFiEsp.h>
constexpr auto data_pin = 4; // S на плате
constexpr auto vPin = 2; // Не - и не S. Питание 5в
constexpr auto dhtType = DHT11; //DHT22 для 22
DHT dht(data_pin, dhtType);
int status = WL_IDLE_STATUS;
int reqCount = 0;
WiFiEspServer server(80);
#ifndef HAVE_HWSERIAL1
#include "SoftwareSerial.h"
SoftwareSerial Serial1(6, 7); // RX, TX
#endif
// изменить
char ssid[] = "Twim"; // your network SSID (name)
char pass[] = "12345678"; // your network password
// изменить ... по надобности
void initDHT(void){
dht.begin();
pinMode(vPin, OUTPUT);
}
void enableDHT(void)
{
digitalWrite(vPin, HIGH);
}
void disableDHT(void)
{
digitalWrite(vPin, LOW);
}
void readDHTAndSendData(WiFiEspClient client)
{
enableDHT();
delay(1000);
float h = dht.readHumidity();
float t = dht.readTemperature();
float f = dht.readTemperature(true);
DynamicJsonDocument doc(128);
if (!isnan(h) && !isnan(t) && !isnan(f)) {
doc["humidity"] = h;
doc["temp"] = t;
doc["tempF"] = f;
} else {
doc["error"] = "Failed to read from DHT sensor";
}
disableDHT(); // исключить нагревание
serializeJson(doc, client);
}
void loop()
{
WiFiEspClient client = server.available();
if (client) {
Serial.println("New client");
String httpRequest = "";
boolean currentLineIsBlank = true;
while (client.connected()) {
if (client.available()) {
char c = client.read();
Serial.write(c);
httpRequest += c;
if (c == '\n' && currentLineIsBlank) {
Serial.println("Sending response");
client.print(
"HTTP/1.1 200 OK\r\n"
"Content-Type: application/json\r\n"
"Connection: close\r\n"
"\r\n");
if (httpRequest.indexOf("GET /temp") != -1) {
// Обработка запроса /temp
readDHTAndSendData(client);
} else if (httpRequest.indexOf("GET /lamp?pin=") != -1) {
// Обработка запроса /lamp?pin=$ID
// код обработки включения светодиоида
//auto data=httpRequest.substring(httpRequest.indexOf("GET /lamp?pin="));
//auto ID = data.toInt();
} else if (httpRequest.indexOf("GET /off?pin=") != -1) {
// Обработка запроса /off?pin=$ID
//auto data=httpRequest.substring(httpRequest.indexOf("GET /lamp?pin="));
//auto ID = data.toInt();
}
break;
}
if (c == '\n') {
currentLineIsBlank = true;
} else if (c != '\r') {
currentLineIsBlank = false;
}
}
}
delay(10);
client.stop();
Serial.println("Client disconnected");
}
}
void setup()
{
Serial1.begin(9600);
Serial.begin(19200);
initDHT();
// Initialize ESP module
WiFi.init(&Serial1);
if (WiFi.status() == WL_NO_SHIELD) {
Serial.println("WiFi shield not present");
while (true);
}
while (status != WL_CONNECTED) {
Serial.print("Attempting to connect to WPA SSID: ");
Serial.println(ssid);
status = WiFi.begin(ssid, pass);
}
Serial.println("You're connected to the network");
printWifiStatus();
server.begin();
}
void printWifiStatus()
{
// print the SSID of the network you're attached to
Serial.print("SSID: ");
Serial.println(WiFi.SSID());
// print your WiFi shield's IP address
IPAddress ip = WiFi.localIP();
Serial.print("IP Address: ");
Serial.println(ip);
// print where to go in the browser
Serial.println();
Serial.print("To see this page in action, open a browser to http://");
Serial.println(ip);
Serial.println();
}
Модули устанавливать: Sketch — include library — manage libraries
В поиске вбиваем нужные.. WifiESP и ArduinoJson с DHT.
На ESP модуле.. При верном подключении загорается красный светодиод.. При передачи данных мигает синий..
Пример Serial Monitor данных:
[WiFiEsp] Connected to Twim
You're connected to the network
SSID: Twim
IP Address: 192.168.238.29
To see this page in action, open a browser to http://192.168.238.29
[WiFiEsp] Server started on port 80
Можно проверять через ARP.. настоящий IP. Может ошибаться… * Результат изменен для примера
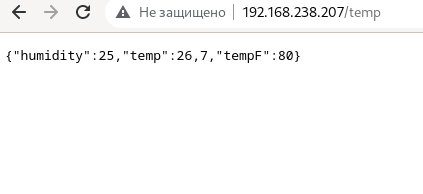